1.
Write a method named
buildTree
that returns a tree using the nodes and references implementation that looks like this: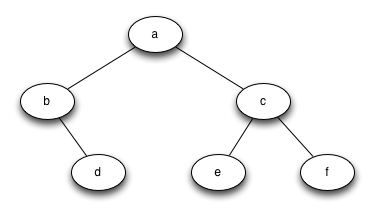
leftChild
and rightChild
will become references to other instances of the BinaryTree
class. For example, when we insert a new left child into the tree, we create another instance of BinaryTree
and modify this.leftChild
in the root to reference the new tree.class BinaryTree<T> {
T key;
BinaryTree<T> leftChild;
BinaryTree<T> rightChild;
public BinaryTree(T rootObject) {
this.key = rootObject;
this.leftChild = null;
this.rightChild = null;
}
// more code here..
}
BinaryTree
class.leftChild
attribute of the root to refer to this new object. The code for insertLeft
is shown in Listing 6.6.3.public void insertLeft(T newNode) {
if (this.leftChild == null) {
this.leftChild = new BinaryTree<T>(newNode);
} else {
BinaryTree<T> newChild = new BinaryTree<T>(newNode);
newChild.leftChild = this.leftChild;
this.leftChild = newChild;
}
}
else
statement on line 4 of Listing 6.6.3.insertRight
must consider a symmetric set of cases. There will either be no right child, or we must insert the node between the root and an existing right child. The insertion code is shown in Listing 6.6.4.public void insertRight(T newNode) {
if (this.rightChild == null) {
this.rightChild = new BinaryTree<T>(newNode);
} else {
BinaryTree<T> newChild = new BinaryTree<T>(newNode);
newChild.rightChild = this.rightChild;
this.rightChild = newChild;
}
}
public T getRootValue() {
return this.key;
}
public void setRootValue(T value) {
this.key = value;
}
public BinaryTree<T> getLeftChild() {
return this.leftChild;
}
public BinaryTree<T> getRightChild() {
return this.rightChild;
}
key
, leftChild
, and rightChild
. Notice that both the left and right children of the root are themselves distinct instances of the BinaryTree
class. As we said in our original recursive definition for a tree, this allows us to treat any child of a binary tree as a binary tree itself.BinaryTree
ClassbuildTree
that returns a tree using the nodes and references implementation that looks like this: