9.2. Using Repetition with Images¶
In Python, the line of code img = Image("cat.jpg")
creates an Image object in the
program that has all of the data from the image cat.jpg and names it with the variable
img
. Once we have done that, we can draw the entire image using lines of code like this:
win = ImageWin(img.getWidth(), img.getHeight()) # Make a window to hold the image
img.draw(win) # draw it in that window
To modify the colors in an image, we need to modify all of its pixels one by one. In an image with 60,000 pixels, we would not want to write a separate line of code for each pixel. What we would like to do is specify what code to run for each of the pixels.
That is exactly what a for
loop does - it takes a list of values and some instructions
and runs the instructions for each one of the values. We can get a list of all the pixels
with the procedure img.getPixels()
.
The program below modifies the cat image to remove all the red from each of its pixels. There are lot of lines in the program below,
Warning
Be patient with program that modify images, they can take a little while to produce their results. Don’t press the “Save and Run” button multiple times or you will just have to wait longer.
If you do hit the button too many times, you can reload the browser window to stop the program.
There are \(200 \times 300 = 60,000\) pixels in the pixelList
. Our for loop takes that giant
list of pixels and one by one, calls the current one p
and then does the body of the loop
with it. After the loop is done, we make a window to display the image in and draw it - but
don’t worry too much about that code - we want to focus on the loop and what it does to each
pixel. This program changes the red value of each pixel to 0 - essentially it removes all the
red from the image, making it look like we are looking through blue-green glass at it.
9.3. A Library of Images¶
The images we work with in this book must be built into the webpage - you can’t use an image
from your own computer. Below are some images that are built into the webpages in this chapter
that we can use. To use a different image, use it’s name in the
command that creates an image img = Image("student1.jpg")
.
Try out one or more of these images by changing line 5 in the program above.
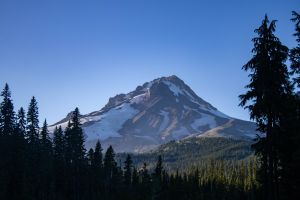
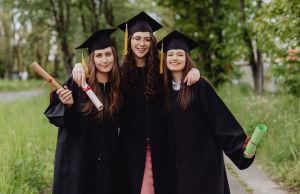
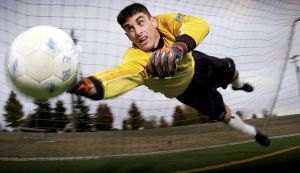
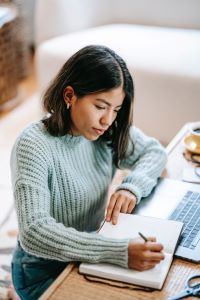
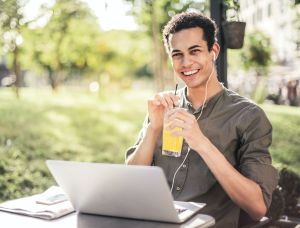
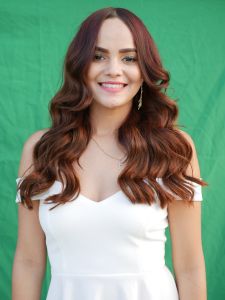
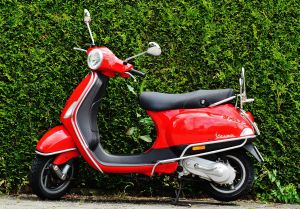
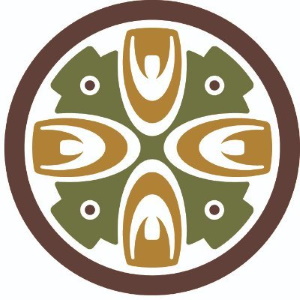
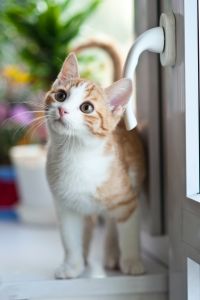
Note
It is also possible to use an image that is on another web page if you know its full address. Doing this would look like:
img = Image("https://shorturl.at/dNUX7")
If you try this, make sure to stick to small images or your program will take a very long time to run.