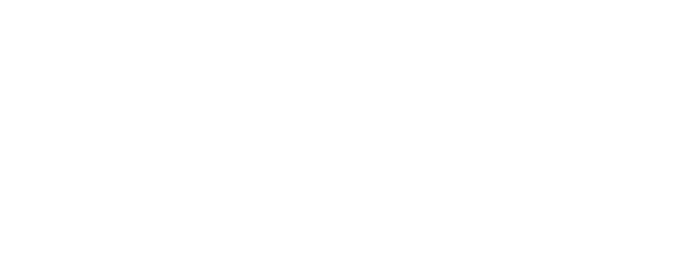
9.5. Introduction to Python¶
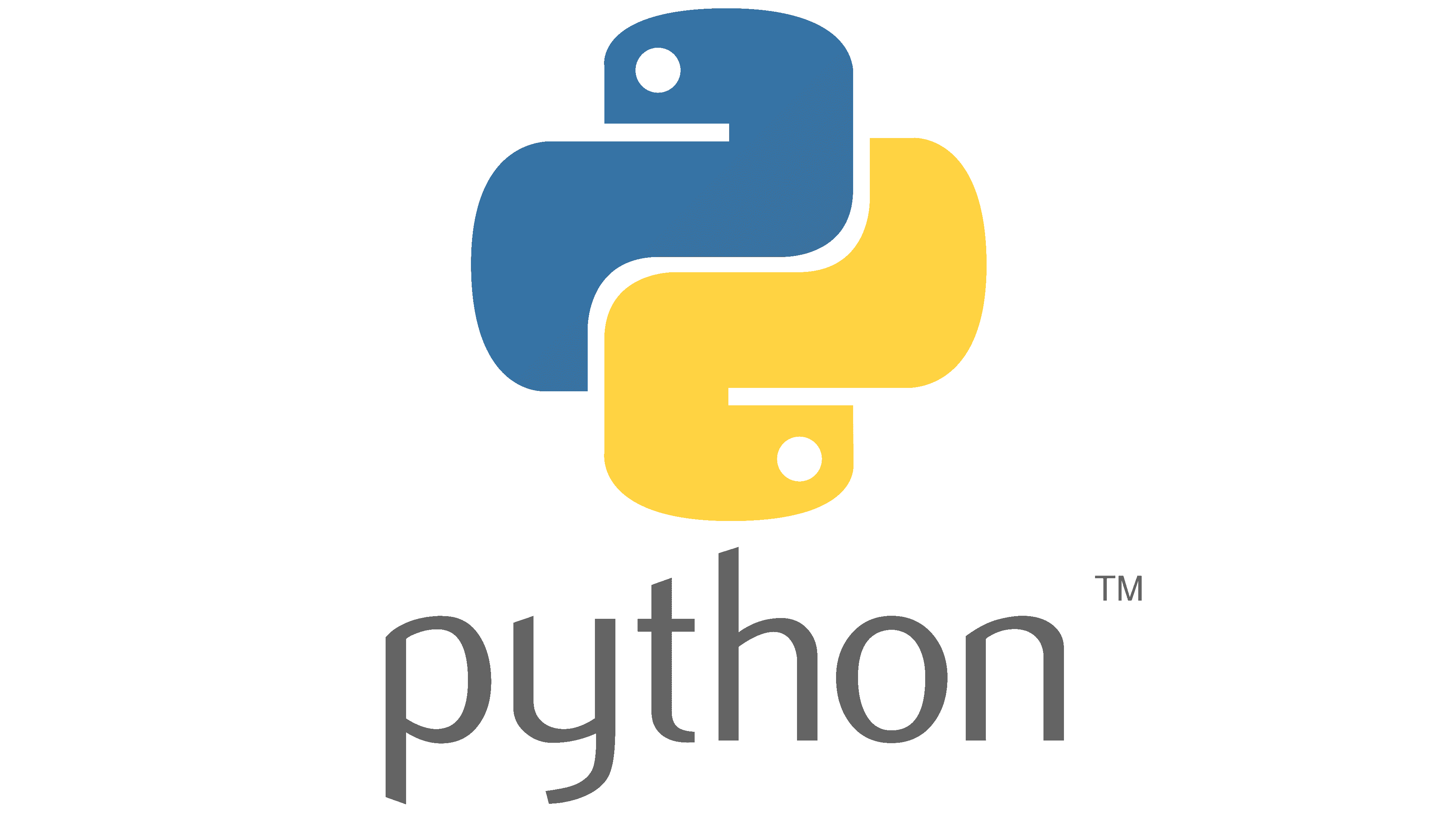
Python is a very popular text-based programming language used in software development, web development, cybersecurity, data analysis, artificial intelligence, etc. Python is a great language for beginners to learn because it is easy to read and understand. Let’s try some Python!
9.5.1. Python Output¶
Python has a built-in function called print
that can be used for output to display text on the screen. The following code will display the text “Hello World!” on the screen. Try it out by clicking the “Run” button. Then, change “World” to your name and run the code again.
Notice that every Python command to the computer must be on a separate line.
Run the following code. Then, change “World” to your name and run the code again.
Drag or click on the blocks you need to move them from the top section into the yellow area to create a print statement. There are extra blocks that you don’t need.
9.5.2. Debugging¶
Syntax errors are bugs or mistakes in the way the code is written. For example, the following code has a syntax error because the quotes and parenthesis are not closed. Try running the code and see what happens. Try fixing the errors to debug the code.
Run the following code. Fix the errors and run again.
9.5.3. Python Variables¶
Variables are names for memory locations to store values. In Python, a variable is created when a value is assigned to it. For example, the following code creates a variable named name
and assigns it the value "Rain"
. Then, it creates a variable named age
and assigns it the value 16
. Multiple values can be printed out separated by commas. The variables, name and age, are never put inside quotes because we do not want to print the variable names; we want to print the values stored in the variables.
name = "Rain"
age = 16
print(name, "is", age, "years old.")
Try changing the variable values in the code below and run it to see what happens.
Try changing “Rain” to your name and 16 to your age in the code below and run it to see what happens.
Drag or click on the blocks you need to move them from the top section into the yellow area to create a print statement with the variable name
. For example, if name = "Alex"
, it will print “Hello Alex!”. There are extra blocks that you don’t need.
9.5.4. Python Input¶
Python has an input function that can be used to get input from the user. The following code will ask the user for their name and then print out a greeting. Try it out by clicking the “Run” button.
Run the following code. Enter your name in the pop up input box and then scroll down to see the output.
Drag or click on the blocks you need to move them from the top section into the yellow area to create a input statement.
9.5.5. Story Project¶
Let’s make a poem or a story using input and variables. Ask the user to input different nouns and verbs, and weave together a story.
Finish the input statements below to ask the user for 2 colors and a food item. Run to see the silly poem. Then, ask the user for more input words and create your own poem or story using the variables in print statements.
9.5.6. If statements¶
If statements are used to make decisions in a program. In Python, the :
is used after if
and else
conditions. The statements under the if clause must all be indented and lined up under the if.
if condition:
# do something
else:
# do something else
The condition in an if statement usually tests a variable with a relation operator such as ==
to see if it is equal to a value or expression, or <
less than, >
, etc.
The following code will ask the user for their age and then print out a message depending on whether they are old enough to drive. Note that the input is converted to a number using the int
function which stands for an integer number. Try it out by clicking the “Run” button.
Run the following code twice trying an age under 16 and one over 16. Try adding extra print statements under the if or else blocks. Make sure you indent and line them up!
The following program could be used at a movie theater to ask for your age to determine whether you are old enough to watch a PG-13 rated movie. The blocks have been mixed up and include extra blocks that aren’t needed in the solution (choose between a and b blocks). Drag the needed blocks from the left and put them in the correct order on the right. Make sure you indent correctly! Click the Check button to check your solution. Click on Help if you get stuck.
9.5.7. Adventure Game Project¶
Let’s create an adventure game with nested if-else statements.
Create an adventure game with nested if-else statements. Fill in the …’s in the print statements and add more if statements for different choices in the adventure.
9.5.8. Python Turtles and Functions¶
Python has a Turtle
library where turtle objects can move around on the screen and draw pictures. We can import the library and set up the drawing space and the turtle with the following code:
from turtle import * # use the turtle library
space = Screen() # create a turtle space
yertle = Turtle() # create a turtle named yertle
The Turtle object yertle
can be used to call functions to move the turtle around the screen. For example, the following code will move the turtle forward 100 pixels, turn it left 90 degrees, and move it forward 100 pixels again. The amount of movement or turning is put in parentheses after the function name. These are called arguments in the function call.
yertle.forward(100) # move forward 100 pixels
yertle.left(90) # turn left 90 degrees
yertle.forward(100) # move forward 100 pixels
Try running the following code to see what happens. Then, try to make the turtle complete the square.
Run the following code. Can you make the turtle complete the square?
9.5.9. Turtle Project¶
Change the code below to draw something with the turtle. The code below also shows how to change colors, fill in colors, lift up the pen, and draw dots. You could draw a flower, a house for the turtle, a smiley face, or anything you want.