1.4. The Python Programming Language¶
The programming language you will be learning is Python. Python is an example of a high-level language; other high-level languages you might have heard of are C++, PHP, and Java.
As you might infer from the name high-level language, there are also low-level languages, sometimes referred to as machine languages or assembly languages. Loosely speaking, computers can only execute programs written in low-level languages. Thus, programs written in a high-level language have to be processed before they can run. This extra processing takes some time, which is a small disadvantage of high-level languages. However, the advantages to high-level languages are enormous.
First, it is much easier to program in a high-level language. Programs written in a high-level language take less time to write, they are shorter and easier to read, and they are more likely to be correct. Second, high-level languages are portable, meaning that they can run on different kinds of computers with few or no modifications. Low-level programs can run on only one kind of computer and have to be rewritten to run on another.
Due to these advantages, almost all programs are written in high-level languages. Low-level languages are used only for a few specialized applications.
Two kinds of programs process high-level languages into low-level languages: interpreters and compilers. An interpreter reads a high-level program and executes it, meaning that it does what the program says. It processes the program a little at a time, alternately reading lines and performing computations.
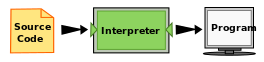
A compiler reads the program and translates it completely before the program starts running. In this case, the high-level program is called the source code, and the translated program is called the object code or the executable. Once a program is compiled, you can execute it repeatedly without further translation. If you make changes to your source code, you need to compile your files into an executable again.

Many modern languages use both processes. They are first compiled into a lower level language, called byte code, and then interpreted by a program called a virtual machine. Python uses both processes, but because of the way programmers interact with it, it is usually considered an interpreted language.
For the core material in this book, you will not need to install or run python natively on your computer. Instead, you’ll be writing simple programs and executing them right in your browser.
At some point, you will find it useful to have a complete python environment, rather than the limited environment available in this online textbook. To do that, you will either install python on your computer so that it can run natively, or use a remote server that provides either a command line shell or a jupyter notebook environment.
1.4.1. ActiveCode in Runestone¶
This book provides two ways to execute Python programs. Both techniques are designed to assist you as you learn the Python programming language. They will help you increase your understanding of how Python programs work.
First, you can write, modify, and execute programs using a unique ActiveCode interpreter that allows you to execute Python code right in the text itself (right from the web browser). Although this is certainly not the way real programs are written, it provides an excellent environment for learning a programming language like Python since you can experiment with the language as you are reading.
Take a look at the ActiveCode interpreter in action. Try pressing the Save & Run button below. (If you are not logged in, it will just say Run.)
Now try modifying the program shown above. First, modify the string (the sentence in quotations) in the
first print statement by changing the word adds to the word multiplies. Now press
Save & Run again. You can see that the result of the program has changed. However, it still prints
“5” as the answer. Modify the second print statement by changing the addition symbol, the
+
, to the multiplication symbol, *
. Press Save & Run again to see the new results.
As the name suggests, Save & Run also saves your latest version of the code, and you can recover it in later sessions when logged in. If not logged in, Run saves versions only until your browser leaves the current web page, and then you lose all modifications.
If you are logged in, when a page first loads, each ActiveCode window will have a Load History button, to the right of the Save & Run button. If you click on it, or if you run any code, that button turns into a slider. If you click on the slider location box, you can use your left and right arrow buttons to switch to other versions you ran. Alternatively you can drag the box on the slider. Now move the slider to see a previously saved version of your code. You can edit or run any version.
1.4.2. CodeLens in Runestone¶
In addition to ActiveCode, you can also execute Python code with the assistance of a unique visualization tool. This tool, known as CodeLens, allows you to control the step by step execution of a program. It also lets you see the values of all variables as they are created and modified. In ActiveCode, the source code executes from beginning to end and you can only see the final result. In CodeLens you can see and control the step by step progress. Note that the red arrow always points to the next line of code that is going to be executed. The light green arrow points to the line that was just executed. Click on the “Show in CodeLens” button in the code example above to make the CodeLens window show up, and then click on the Forward button a few times to step through the execution.
Sometimes, we will present code examples explicitly in a CodeLens window in the textbook, as below. When we do, think of it as an encouragement to use the CodeLens features to step through the execution of the program to understand how the code is executed. This is really important to to and it will help you learn to ‘Think Like a Computer’.
Activity: CodeLens 1.4.2.1 (clens_first_example)
Check your understanding
- the instructions in a program, written in a high-level language.
- If the instructions are stored in a file, it is called the source code file.
- the language that you are programming in (e.g. Python).
- This language is simply called the programming language, or simply the language. Programs are written in this language.
- the environment/tool in which you are programming.
- The environment may be called the IDE, or Integrated Development Environment, though not always.
- the number (or “code”) that you must input at the top of each program to tell the computer how to execute your program.
- There is no such number that you must type in at the start of your program.
Source code is another name for:
- It is high-level if you are standing and low-level if you are sitting.
- In this case high and low have nothing to do with altitude.
- It is high-level if you are programming for a computer and low-level if you are programming for a phone or mobile device.
- High and low have nothing to do with the type of device you are programming for. Instead, look at what it takes to run the program written in the language.
- It is high-level if the program must be processed before it can run, and low-level if the computer can execute it without additional processing.
- Python is a high level language but must be interpreted into machine code (binary) before it can be executed.
- It is high-level if it easy to program in and is very short; it is low-level if it is really hard to program in and the programs are really long.
- While it is true that it is generally easier to program in a high-level language and programs written in a high-level language are usually shorter, this is not always the case.
What is the difference between a high-level programming language and a low-level programming language?
- 1 = a process, 2 = a function
- Compiling is a software process, and running the interpreter is invoking a function, but how is a process different than a function?
- 1 = translating an entire book, 2 = translating a line at a time
- Compilers take the entire source code and produce object code or the executable and interpreters execute the code line by line.
- 1 = software, 2 = hardware
- Both compilers and interpreters are software.
- 1 = object code, 2 = byte code
- Compilers can produce object code or byte code depending on the language. An interpreter produces neither.
Pick the best replacements for 1 and 2 in the following sentence: When comparing compilers and interpreters, a compiler is like 1 while an interpreter is like 2.
- save programs and reload saved programs.
- You can (and should) save the contents of the ActiveCode window.
- type in Python source code.
- You are not limited to running the examples that are already there. Try adding to them and creating your own.
- execute Python code right in the text itself within the web ..
- The ActiveCode interpreter will allow you type Python code into the textbox and then you can see it execute as the interpreter interprets and executes the source code.
- receive a yes/no answer about whether your code is correct or not.
- Although you can (and should) verify that your code is correct by examining its output, ActiveCode will not directly tell you whether you have correctly implemented your program.
The ActiveCode interpreter allows you to (select all that apply):
- measure the speed of a program’s execution.
- In fact, CodeLens steps through each line one by one as you click, which is MUCH slower than the Python interpreter.
- control the step by step execution of a program.
- By using CodeLens, you can control the execution of a program step by step. You can even go backwards!
- write and execute your own Python code.
- Codelens works only for the pre-programmed examples.
- execute the Python code that is in codelens.
- By stepping forward through the Python code in CodeLens, you are executing the Python program.
CodeLens allows you to (select all that apply):